演示图
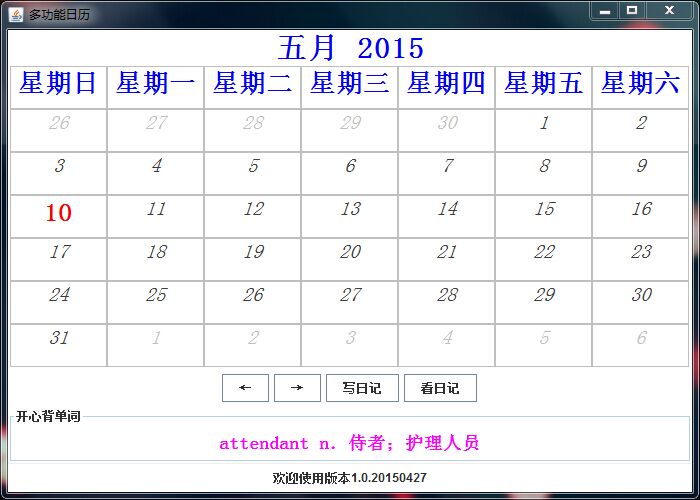
演示图
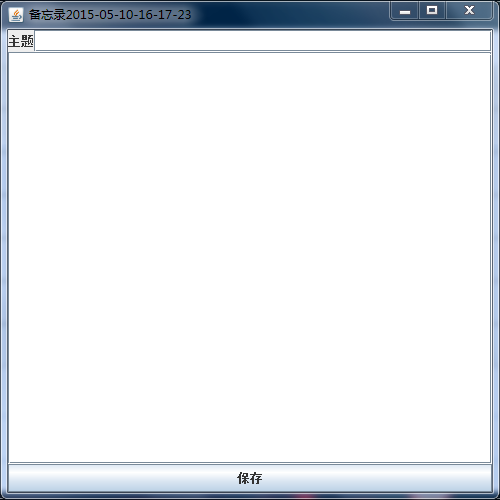
CalendarApp.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
|
package calenda; import java.awt.BorderLayout; import java.awt.Color; import java.awt.FlowLayout; import java.awt.Font; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; import java.io.File; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; import java.util.GregorianCalendar; import java.util.Locale; import javax.swing.JApplet; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.SwingUtilities; import javax.swing.border.TitledBorder; public class CalendarApp extends JFrame{ private CalendarPanel calendarPanel= new CalendarPanel(); private static JLabel jlblLearn= new JLabel( "开始准备记单词" ); private String versionID= "欢迎使用版本1.0.20150427" ; private JLabel jlblVersionID= new JLabel(versionID); private JButton jbtPrior= new JButton( "←" ); private JButton jbtNext= new JButton( "→" ); private JButton jbtDiary= new JButton( "写日记" ); private JButton jbtScanDiary= new JButton( "看日记" ); private static int year; public void init() { year=calendarPanel.getYear(); calendarPanel.setBackground(Color.WHITE); /** * 添加功能性按钮到日历面板下方 */ JPanel jpButtons= new JPanel( new FlowLayout()); //设置按钮背景色为白色 jbtPrior.setBackground(Color.WHITE); jbtNext.setBackground(Color.WHITE); jbtDiary.setBackground(Color.WHITE); jbtScanDiary.setBackground(Color.WHITE); /* * 给四个按钮添加鼠标事件,使其更加炫酷 */ //一、后退按钮 jbtPrior.addMouseListener(new MouseListener(){ @Override public void mouseClicked(MouseEvent arg0) { // TODO Auto-generated method stub } @Override public void mouseEntered(MouseEvent arg0) { // TODO Auto-generated method stub jbtPrior.setForeground(Color.GREEN); } @Override public void mouseExited(MouseEvent arg0) { // TODO Auto-generated method stub jbtPrior.setForeground(Color.BLACK); } @Override public void mousePressed(MouseEvent arg0) { // TODO Auto-generated method stub } @Override public void mouseReleased(MouseEvent arg0) { // TODO Auto-generated method stub } }); //二、前进按钮 jbtNext.addMouseListener(new MouseListener(){ @Override public void mouseClicked(MouseEvent arg0) { // TODO Auto-generated method stub } @Override public void mouseEntered(MouseEvent arg0) { // TODO Auto-generated method stub jbtNext.setForeground(Color.GREEN); } @Override public void mouseExited(MouseEvent arg0) { // TODO Auto-generated method stub jbtNext.setForeground(Color.BLACK); } @Override public void mousePressed(MouseEvent arg0) { // TODO Auto-generated method stub } @Override public void mouseReleased(MouseEvent arg0) { // TODO Auto-generated method stub } }); //三、写日记按钮 jbtDiary.addMouseListener(new MouseListener(){ @Override public void mouseClicked(MouseEvent arg0) { // TODO Auto-generated method stub } @Override public void mouseEntered(MouseEvent arg0) { // TODO Auto-generated method stub jbtDiary.setForeground(Color.GREEN); } @Override public void mouseExited(MouseEvent arg0) { // TODO Auto-generated method stub jbtDiary.setForeground(Color.BLACK); } @Override public void mousePressed(MouseEvent arg0) { // TODO Auto-generated method stub } @Override public void mouseReleased(MouseEvent arg0) { // TODO Auto-generated method stub } }); //四、看日记按钮 jbtScanDiary.addMouseListener(new MouseListener(){ @Override public void mouseClicked(MouseEvent arg0) { // TODO Auto-generated method stub } @Override public void mouseEntered(MouseEvent arg0) { // TODO Auto-generated method stub jbtScanDiary.setForeground(Color.GREEN); } @Override public void mouseExited(MouseEvent arg0) { // TODO Auto-generated method stub jbtScanDiary.setForeground(Color.BLACK); } @Override public void mousePressed(MouseEvent arg0) { // TODO Auto-generated method stub } @Override public void mouseReleased(MouseEvent arg0) { // TODO Auto-generated method stub } }); jpButtons.add(jbtPrior); jpButtons.add(jbtNext); jpButtons.add(jbtDiary); jpButtons.add(jbtScanDiary); jpButtons.setBackground(Color.WHITE); /** * 添加日历主要组件 */ JPanel jpCalendar=new JPanel(new BorderLayout()); jpCalendar.add(calendarPanel,BorderLayout.CENTER); jpCalendar.add(jpButtons,BorderLayout.SOUTH); /** * 添加背单词模块 */ JPanel jpLearn=new JPanel(new FlowLayout()); jpLearn.setBorder(new TitledBorder("开心背单词")); jpLearn.add(jlblLearn); jpLearn.setBackground(Color.WHITE); /** * 添加版本号信息 */ JPanel jpVersionID=new JPanel(new FlowLayout()); Font font=new Font("宋体",Font.PLAIN,4); jpVersionID.setFont(font); jpVersionID.add(jlblVersionID); jpVersionID.setBackground(Color.WHITE); /** * 容器面板,合并记单词与版本号模块 */ JPanel jpBelow= new JPanel( new BorderLayout( 2 , 1 )); jpBelow.add(jpLearn,BorderLayout.CENTER); jpBelow.add(jpVersionID,BorderLayout.SOUTH); this .add(jpCalendar,BorderLayout.CENTER); this .add(jpBelow,BorderLayout.SOUTH); this .setBackground(Color.WHITE); this .setSize( 700 , 500 ); this .setLocationRelativeTo( null ); this .setTitle( "多功能日历" ); this .setDefaultCloseOperation(EXIT_ON_CLOSE); this .setVisible( true ); jbtScanDiary.addActionListener( new ActionListener() { @Override public void actionPerformed(ActionEvent e) { new Thread( new thread_scanDiary()).start(); } }); jbtDiary.addActionListener( new ActionListener() { @Override public void actionPerformed(ActionEvent e) { //获取本地系统时间 SimpleDateFormat df = new SimpleDateFormat( "yyyy-MM-dd-HH-mm-ss" ); //设置日期格式 String time=df.format( new Date()); new Thread( new thread_keepDiary(time)).start(); } }); jbtPrior.addActionListener( new ActionListener() { @Override public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stub int currentMonth=calendarPanel.getMonth(); if (currentMonth== 0 ) { calendarPanel.setYear(year); year--; } calendarPanel.setMonth((currentMonth- 1 )% 12 ); } }); jbtNext.addActionListener( new ActionListener() { @Override public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stub int currentMonth=calendarPanel.getMonth(); if (currentMonth== 11 ) { calendarPanel.setYear(++year); } calendarPanel.setMonth((currentMonth+ 1 )% 12 ); } }); } public static void main(String[] args) throws InterruptedException { // TODO Auto-generated method stub SwingUtilities.invokeLater( new Runnable(){ @Override public void run() { // TODO Auto-generated method stub new CalendarApp().init(); new Thread( new thread_showEnglish(jlblLearn)).start(); } }); } } |
CalendarPanel.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
|
/** * 本程序功能是进行日历主面板布局 */ package calenda; import java.awt.BorderLayout; import java.awt.Color; import java.awt.Font; import java.awt.GridLayout; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; import java.text.DateFormatSymbols; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.GregorianCalendar; import java.util.Locale; import javax.swing.*; import javax.swing.border.LineBorder; public class CalendarPanel extends JPanel { /** * 声明数据变量 */ private static final long serialVersionUID = 1L; private JLabel jlblHeader= new JLabel( " " ,JLabel.CENTER); private JLabel[] jlblDay= new JLabel[ 49 ]; private Calendar calendar= new GregorianCalendar(); private int year0=calendar.get(Calendar.YEAR); private int month0=calendar.get(Calendar.MONTH); private int day0=calendar.get(Calendar.DAY_OF_MONTH); private int month; private int year; private int day; private JPanel jpDays= new JPanel( new GridLayout( 0 , 7 )); Font font1= new Font( "宋体" ,Font.ITALIC, 20 ); Font font2= new Font( "宋体" ,Font.BOLD, 26 ); Font font3= new Font( "宋体" ,Font.BOLD, 30 ); public CalendarPanel() { //设置日历头部件以及日期标签的背景色为白色 jlblHeader.setBackground(Color.WHITE); jpDays.setBackground(Color.WHITE); //声明每个标签 for ( int i= 0 ;i< 49 ;i++) { jlblDay[i]= new JLabel(); jlblDay[i].setBorder( new LineBorder(Color.LIGHT_GRAY, 1 )); jlblDay[i].setHorizontalAlignment(JLabel.RIGHT); jlblDay[i].setVerticalAlignment(JLabel.TOP); } calendar= new GregorianCalendar(); month=calendar.get(Calendar.MONTH); year=calendar.get(Calendar.YEAR); day=calendar.get(Calendar.DATE); //更新日历 updateCalendar(); showHeader(); showDays(); //添加到主面板 this .setLayout( new BorderLayout()); this .add(jlblHeader, BorderLayout.NORTH); this .add(jpDays, BorderLayout.CENTER); } private void showHeader() { SimpleDateFormat sdf= new SimpleDateFormat( "MMMM yyyy" ,Locale.CHINA); String header=sdf.format(calendar.getTime()); jlblHeader.setText(header); jlblHeader.setForeground(Color.BLUE); jlblHeader.setFont(font3); } private void showDayNames() { DateFormatSymbols dfs= new DateFormatSymbols(Locale.CHINA); String dayNames[]=dfs.getWeekdays(); for ( int i= 0 ;i< 7 ;i++) { jlblDay[i].setText(dayNames[i+ 1 ]); jlblDay[i].setForeground(Color.BLUE); jlblDay[i].setHorizontalAlignment(JLabel.CENTER); jlblDay[i].setFont(font2); jpDays.add(jlblDay[i]); } } public void showDays() { jpDays.removeAll(); showDayNames(); int startingDayOfMonth=calendar.get(Calendar.DAY_OF_WEEK); Calendar cloneCalendar=(Calendar)calendar.clone(); cloneCalendar.add(Calendar.DATE, - 1 ); int daysInPrecedingMonth=cloneCalendar.getActualMaximum(Calendar.DAY_OF_MONTH); for ( int i= 0 ;i<startingDayOfMonth- 1 ;i++) { jlblDay[i+ 7 ].setForeground(Color.LIGHT_GRAY); jlblDay[i+ 7 ].setHorizontalAlignment(JLabel.CENTER); jlblDay[i+ 7 ].setText(daysInPrecedingMonth-startingDayOfMonth+ 2 +i+ "" ); jlblDay[i+ 7 ].setFont(font1); jpDays.add(jlblDay[i+ 7 ]); } int daysInCurrentMonth=calendar.getActualMaximum(Calendar.DAY_OF_MONTH); for ( int i= 1 ;i<=daysInCurrentMonth;i++) { if (i==day0&&year==year0&&month==month0) { jlblDay[i- 2 +startingDayOfMonth+ 7 ].setForeground(Color.red); jlblDay[i- 2 +startingDayOfMonth+ 7 ].setHorizontalAlignment(JLabel.CENTER); jlblDay[i- 2 +startingDayOfMonth+ 7 ].setText(i+ "" ); jlblDay[i- 2 +startingDayOfMonth+ 7 ].setFont(font2); jpDays.add(jlblDay[i- 2 +startingDayOfMonth+ 7 ]); } else { jlblDay[i- 2 +startingDayOfMonth+ 7 ].setForeground(Color.darkGray); jlblDay[i- 2 +startingDayOfMonth+ 7 ].setHorizontalAlignment(JLabel.CENTER); jlblDay[i- 2 +startingDayOfMonth+ 7 ].setText(i+ "" ); jlblDay[i- 2 +startingDayOfMonth+ 7 ].setFont(font1); jpDays.add(jlblDay[i- 2 +startingDayOfMonth+ 7 ]); } } int j= 1 ; for ( int i=daysInCurrentMonth- 1 +startingDayOfMonth+ 7 ;i% 7 != 0 ;i++) { jlblDay[i].setForeground(Color.LIGHT_GRAY); jlblDay[i].setHorizontalAlignment(JLabel.CENTER); jlblDay[i].setText(j++ + "" ); jlblDay[i].setFont(font1); jpDays.add(jlblDay[i]); } jpDays.repaint(); } public void updateCalendar() { calendar.set(Calendar.YEAR, year); calendar.set(Calendar.MONTH, month); calendar.set(Calendar.DATE, 1 ); month=calendar.get(Calendar.MONTH); year=calendar.get(Calendar.YEAR); day=calendar.get(Calendar.DATE); } public int getMonth() { return month; } public int getYear() { return year; } public void setMonth( int month) { this .month=month; updateCalendar(); showHeader(); showDays(); } public void setYear( int year) { this .year=year; updateCalendar(); showHeader(); showDays(); } } |
Diary.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
|
/** * 本程序为日记类 * 最后修改日期为2015-4-27 */ package calenda; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.io.Serializable; import javax.swing.JOptionPane; public class Diary implements Serializable { /** * 数据域 */ //私有变量 private String filename; private String theme; private String content; //含参构造方法 public Diary(String filename,String theme,String content) { if (theme.length()== 0 ) { JOptionPane.showMessageDialog( null , "无论心情如何,总得有个主题吧!" ); } else if (content.length()== 0 ) { JOptionPane.showMessageDialog( null , "把开心的不开心的都尽情写下吧!" ); } else { File CalendarDiaryFile= new File( "C:/Calendar/Diary" ); if (!CalendarDiaryFile.exists()) { CalendarDiaryFile.mkdirs(); } this .filename= "C:/Calendar/Diary/" +filename+ ".dat" ; this .theme=theme; this .content=content; } } public void write() throws Exception { File file= new File(filename); FileOutputStream fos = new FileOutputStream(file); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject( this ); oos.close(); } public Diary read() throws Exception { File file= new File(filename); FileInputStream fis= new FileInputStream(file); ObjectInputStream ois = new ObjectInputStream(fis); Diary d=(Diary) ois.readObject(); ois.close(); return d; } /** * 变量get()方法 * @return */ public String getTheme() { return this .theme; } public String getContent() { return this .content; } /** * 变量set()方法 * @param comment */ } |
thread_keepDiary.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
|
/** * 备忘录线程 * 用于单击标签时可以存储日记 * 目前只考虑年月日时间点,不标记具体时间点 */ package calenda; import java.awt.BorderLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.File; import java.nio.file.Files; import javax.swing.*; public class thread_keepDiary extends JFrame implements Runnable { private JFrame jf= this ; private JTextArea jta= new JTextArea(); private JButton jbtSave= new JButton( "保存" ); private JLabel jlblTitle= new JLabel( "主题" ); private JTextField jtfTitle= new JTextField( 16 ); private String id; public thread_keepDiary(String time) { JPanel jpTitle= new JPanel(); jpTitle.setLayout( new BorderLayout()); jpTitle.add(jlblTitle, BorderLayout.WEST); jpTitle.add(jtfTitle,BorderLayout.CENTER); jta.setLineWrap( true ); jta.setWrapStyleWord( true ); JScrollPane jsp= new JScrollPane(jta); jsp.setHorizontalScrollBarPolicy(JScrollPane.HORIZONTAL_SCROLLBAR_NEVER); this .id=time; jbtSave.addActionListener( new ActionListener() { @Override public void actionPerformed(ActionEvent arg0) { // TODO Auto-generated method stub String theme=jtfTitle.getText().trim(); String content=jta.getText(); Diary d_today= new Diary(id,theme,content); try { d_today.write(); JOptionPane.showMessageDialog( null , "保存成功!" ); jf.dispose(); } catch (Exception e) { e.printStackTrace(); } } }); this .setTitle( "备忘录" +id); this .add(jsp,BorderLayout.CENTER); this .add(jpTitle, BorderLayout.NORTH); this .add(jbtSave, BorderLayout.SOUTH); this .setSize( 500 , 500 ); this .setLocationRelativeTo( null ); this .setDefaultCloseOperation(DISPOSE_ON_CLOSE); } @Override public void run() { this .setVisible( true ); } } |
thread_mottoSparkle.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
package calenda; import javax.swing.JLabel; public class thread_mottoSparkle implements Runnable{ private JLabel jlbl; public thread_mottoSparkle(JLabel jlbl) { this .jlbl=jlbl; } @Override public void run() { String content=jlbl.getText(); int L=content.length(); int index= 0 ; while ( true ) { jlbl.setText(content.substring( 0 , index)); try { Thread.sleep( 250 ); } catch (Exception ex) { ex.printStackTrace(); } index++; if (index==(L+ 1 )) index= 0 ; } } } |
thread_scanDiary.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
|
/** * 查看日记线程编写 */ package calenda; import java.awt.BorderLayout; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.File; import java.io.FileInputStream; import java.io.ObjectInputStream; import javax.swing.*; import javax.swing.table.DefaultTableCellRenderer; import javax.swing.table.DefaultTableModel; import javax.swing.table.JTableHeader; public class thread_scanDiary extends JFrame implements Runnable{ /** * 声明变量区 */ private static final long serialVersionUID = 1L; //日历数据库存放路径 private String path= "C:/Calendar/Diary" ; //日历总个数 private static int num; //声明日历文件 private File file; private File[] Diary; //声明JTable模型 private JTable jtable; //声明格言面板及标签及内容等 private JPanel jpMotto= new JPanel(); private JLabel jlblMotto= new JLabel(); private Font font= new Font( "宋体" ,Font.ITALIC, 20 ); private String wish= "唯有专注,才能让自己成功。" ; //增加弹出式菜单2015-4-26 private JPopupMenu jPopupMenu1= new JPopupMenu(); //声明菜单 private JMenuItem jmiScan= new JMenuItem( "查看" ); private JMenuItem jmiDelete= new JMenuItem( "删除" ); private JMenuItem jmiComment= new JMenuItem( "评论" ); @Override public void run() { //尝试弹出式菜单增加子菜单 jmiScan.setForeground(Color.RED); jmiDelete.setForeground(Color.RED); jmiComment.setForeground(Color.RED); jPopupMenu1.add(jmiScan); jPopupMenu1.addSeparator(); jPopupMenu1.add(jmiDelete); jPopupMenu1.addSeparator(); jPopupMenu1.add(jmiComment); /** * 智能获取文件列表 */ file = new File(path); Diary=file.listFiles(); num=Diary.length; String[] head={ " 时间 " , " 主题 " }; Object[][] diary= new Object[num][ 2 ]; for ( int i= 0 ;i<num;i++) { try { String time=Diary[i].getName().replaceFirst( ".dat" , "" ); FileInputStream fis= new FileInputStream(Diary[i]); ObjectInputStream ois = new ObjectInputStream(fis); Diary d=(Diary) ois.readObject(); ois.close(); String theme=d.getTheme(); diary[i][ 0 ]=time; diary[i][ 1 ]=theme; } catch (Exception ex) { ex.printStackTrace(); } } /** * 格言面板取 */ jlblMotto.setText(wish); jlblMotto.setFont(font); jlblMotto.setForeground(Color.RED); jpMotto.add(jlblMotto); jpMotto.setBackground(Color.WHITE); /** * 日历列表面板区 */ final DefaultTableModel tableModel= new DefaultTableModel(diary,head); jtable= new JTable(tableModel); jtable.setBackground(Color.WHITE); jtable.setRowHeight( 30 ); jtable.setDoubleBuffered( false ); jtable.setComponentPopupMenu(jPopupMenu1); jtable.setSelectionMode(ListSelectionModel.SINGLE_SELECTION); DefaultTableCellRenderer tcr = new DefaultTableCellRenderer(); // 设置table内容居中 tcr.setHorizontalAlignment(SwingConstants.CENTER); // 这句和上句作用一样 jtable.setDefaultRenderer(Object. class , tcr); JScrollPane jsp= new JScrollPane(jtable); /** * 弹出式菜单事件监听器编写 */ //查看菜单 jmiScan.addActionListener( new ActionListener(){ @Override public void actionPerformed(ActionEvent e) { if (jtable.getSelectedRow()>= 0 ) { int index=jtable.getSelectedRow(); String filename= "C:/Calendar/Diary/" +Diary[index].getName(); File file= new File(filename); try { FileInputStream fis= new FileInputStream(file); ObjectInputStream ois = new ObjectInputStream(fis); Diary d=(Diary) ois.readObject(); ois.close(); JFrame jf= new JFrame(); JTextArea jta= new JTextArea(); JLabel jlblTitle= new JLabel( "主题" ); JTextField jtfTitle= new JTextField( 16 ); JPanel jpTitle= new JPanel(); jpTitle.setLayout( new BorderLayout()); jpTitle.add(jlblTitle, BorderLayout.WEST); jpTitle.add(jtfTitle,BorderLayout.CENTER); jta.setLineWrap( true ); jta.setWrapStyleWord( true ); JScrollPane jsp= new JScrollPane(jta); jsp.setHorizontalScrollBarPolicy(JScrollPane.HORIZONTAL_SCROLLBAR_NEVER); jtfTitle.setText(d.getTheme()); jta.setText(d.getContent()); jtfTitle.setEditable( false ); jta.setEditable( false ); jf.setTitle( "日记 " +Diary[index].getName().replaceFirst( ".dat" , "" )); jf.add(jsp,BorderLayout.CENTER); jf.add(jpTitle, BorderLayout.NORTH); jf.setSize( 400 , 400 ); jf.setLocationRelativeTo( null ); jf.setDefaultCloseOperation(DISPOSE_ON_CLOSE); jf.setVisible( true ); } catch (Exception ex) { ex.printStackTrace(); } } else { JOptionPane.showMessageDialog( null , "请先选中一个日记!" ); } } }); //删除菜单 jmiDelete.addActionListener( new ActionListener(){ @Override public void actionPerformed(ActionEvent e) { if (jtable.getSelectedRow()>= 0 ) { int index=jtable.getSelectedRow(); String filename= "C:/Calendar/Diary/" +Diary[index].getName(); File file= new File(filename); int option=JOptionPane.showConfirmDialog( null , "你确定要删除日记" +Diary[index].getName()+ "?" ); if (option==JOptionPane.YES_OPTION) { file.delete(); tableModel.removeRow(index); SwingUtilities.updateComponentTreeUI(jtable); JOptionPane.showMessageDialog( null , "删除成功!" ); } else { } } else { JOptionPane.showMessageDialog( null , "请先选中一个日记!" ); } } }); /** * 主框架布局 */ this .add(jsp,BorderLayout.CENTER); this .add(jpMotto, BorderLayout.SOUTH); this .setSize( 600 , 500 ); this .setDefaultCloseOperation(DISPOSE_ON_CLOSE); this .setLocationRelativeTo( null ); this .setTitle( "日记列表" ); this .setVisible( true ); new Thread( new thread_mottoSparkle(jlblMotto)).start(); } } |
thread_showEnglish.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
|
package calenda; import java.awt.Color; import java.awt.Font; import java.io.BufferedReader; import java.io.FileReader; import java.io.LineNumberReader; import java.security.SecureRandom; import java.util.Random; import javax.swing.JLabel; public class thread_showEnglish implements Runnable { private static JLabel jlbl= new JLabel(); Font font= new Font( "���ו" ,Font.BOLD, 18 ); public thread_showEnglish(JLabel jlbl) { this .jlbl=jlbl; jlbl.setForeground(Color.MAGENTA); jlbl.setFont(font); } @Override public void run() { // TODO Auto-generated method stub int count= 0 ; try { String file= "C:/Calendar/Learning/english_word.txt" ; BufferedReader input = new BufferedReader( new FileReader(file)); while (input.readLine()!= null ) { count++; } input.close(); int [] word = new int [count]; SecureRandom random = new SecureRandom(); for ( int i= 0 ;i<count;i++) { word[i]=random.nextInt(count); } int index= 0 ; Thread.sleep( 1000 ); while ( true ) { BufferedReader input1 = new BufferedReader( new FileReader(file)); String content= "" ; int line= 0 ; while ((content=input1.readLine())!= null ) { if (line==word[index]) { jlbl.setText(content); } line++; } if (index==count- 1 ) index= 0 ; else index++; Thread.sleep( 3500 ); } } catch (Exception ex) { ex.printStackTrace(); } } } |
以上所述就是本文的全部内容了,希望大家能够喜欢。